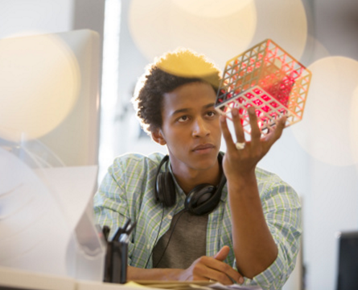
Read Time:6 Minute, 17 Second
Introduction
- MVC is a framework for building web applications with the MVC design.
Now what is MVC?
- MVC is a model-view-control design that seprates buisness logics ,UI design and user interaction.
- Model deals with the business layer that is classes and the properties.
- View is the part that deals with the display of data on the screen.
- Controller interacts with the view and gets the user inputs from view.
- Controller sends input to the model and in return, model sends output to the controller after performing actions.
- Controller then displays the output on screen.
- In traditional webform pattern code and design file is same and if we place the control on the design page that reflects in the code file also.
- In Mvc design this file system is replaced.Here we put all the logic in one file called controller.From one controller we can call different UI.
- In controller there is ActionResult that returns action against the ActionResult.
- There is a view created against the ActionResult with the same name as the action result.
- If we created the view against an ActionResult then in the Views folder a folder is created with the same name as of controller containing the views of that controller only.
Start by creating the project
- Open the VS create new project,find ASP.NET MVC 4 Web Application.
- After that a window appears select Internet Application and click OK.
- Mvc project will be created.
- SolutionExplorer shows many folders.
- Here in the solution explorer every folder has unique meaning.
- Properties folder contains the version information and some other information about the project.
- References store the refrence dlls required in the project.
- App_Start contains the configuration files.
- Content folder contain the css files.
- Controllers folder contain the controllers for code.
- Models folder contain the classes for buisness logics.
- Scripts contain the javascript files.
- Views folder contains views for the controllers.
Download CSS and JS files for bootstrap.
- Go to the References folder right click on the folder >Find>Manage NuGet Packages>Click on the option.
- Install bootstrap package.
- Bootstrap is installed automatically and css files are saved in content folder and js files are saved in script folder.
Now register css and scripts for entire project.
- Go to the App_Start folder > Open BundleConfig.cs,
- Delete the code in the RegisterBundles method and replace with the given code.
public class BundleConfig { public static void RegisterBundles(BundleCollection bundles) { StyleBundle(bundles); ScriptBundle(bundles); } public static void StyleBundle(BundleCollection bundles) { bundles.Add(new StyleBundle("~/css") .Include("~/Content/bootstrap.css")); } public static void ScriptBundle(BundleCollection bundles) { bundles.Add(new ScriptBundle("~/js") .Include("~/Scripts/jquery-{version}.js") .Include("~/Scripts/bootstrap.js")); } }
- StyleBundle method register the css files.
- ScriptBundle method register the js files.
- Check the path of the files.
- ~/css and ~/js are the root path for css and js files.
- Call the both methods in the RegisterBundle method.
- Check the path of the files carefully.
Now create the layout page for the entire project.
- Go to the views folder find shared folder inside views folder.
- Open _Layout.cshtml present in shared folder.
- Write below given code,
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <title>@ViewBag.Title - My ASP.NET MVC Application</title> <link href="~/favicon.ico" rel="shortcut icon" type="image/x-icon" /> <meta name="viewport" content="width=device-width" /> @Styles.Render("~/css") </head> <body> <nav class="navbar navbar-inverse"> <div class="container-fluid"> <!-- Brand and toggle get grouped for better mobile display --> <div class="navbar-header"> <button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#bs-example-navbar-collapse-1" aria-expanded="false"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand">Mvc App</a> </div> <!-- Collect the nav links, forms, and other content for toggling --> <div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1"> <ul class="nav navbar-nav"> <li class="active"><a href="#">1st LINK</a></li> <li><a href="#">2nd Link</a></li> <li class="dropdown"> <a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false">Link Dropdown<span class="caret"></span></a> <ul class="dropdown-menu"> <li><a href="#">SubLink1</a></li> <li><a href="#">SubLink2</a></li> <li><a href="#">SubLink3</a></li> <li role="separator" class="divider"></li> <li><a href="#">SubLink4</a></li> <li role="separator" class="divider"></li> <li><a href="#">SubLink5</a></li> </ul> </li> </ul> <ul class="nav navbar-nav navbar-right"> <li><a href="#">Right side links</a></li> <li class="dropdown"> <a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false">Link Dropdown<span class="caret"></span></a> <ul class="dropdown-menu"> <li><a href="#">1st Link</a></li> <li><a href="#">2nd Link</a></li> <li><a href="#">3rd Link</a></li> <li role="separator" class="divider"></li> <li><a href="#">4th Link</a></li> </ul> </li> </ul> </div> <!-- /.navbar-collapse --> </div> <!-- /.container-fluid --> </nav> <div class="container"> @RenderBody() </div> @Scripts.Render("~/js") @RenderSection("scripts", required: false) </body> </html>
- In the code given above we define the layout for entire project.
- @Styles.Render(“~/css”) this is used to render the css files that included in the bundleConfig.cs file.
- In the body tag nav bar is placed that is used to place the links for the page redirection.
- This layout of bootstrap is fully responsive.
- @RenderBody() is used to render the content on the page.
- @Scripts.Render(“~/js”) include all the js files that included in the BundleConfig.cs file.
- Now HomeController is created automatically inside the controllers folder.
- View against the ActionResult is also created automatically.
- Now inside the views folder find folder named Home ,find Index.cshtml view inside the Home folder.
- Replace the code with the below given code.
@{ ViewBag.Title = "Home Page"; } <h1>Your 1st MVC app created.</h1><br /> <h1><a href="">Click here next part is pretty intresting.</a></h1>
- Now run the application.Result will look like this.
Start with your own project.