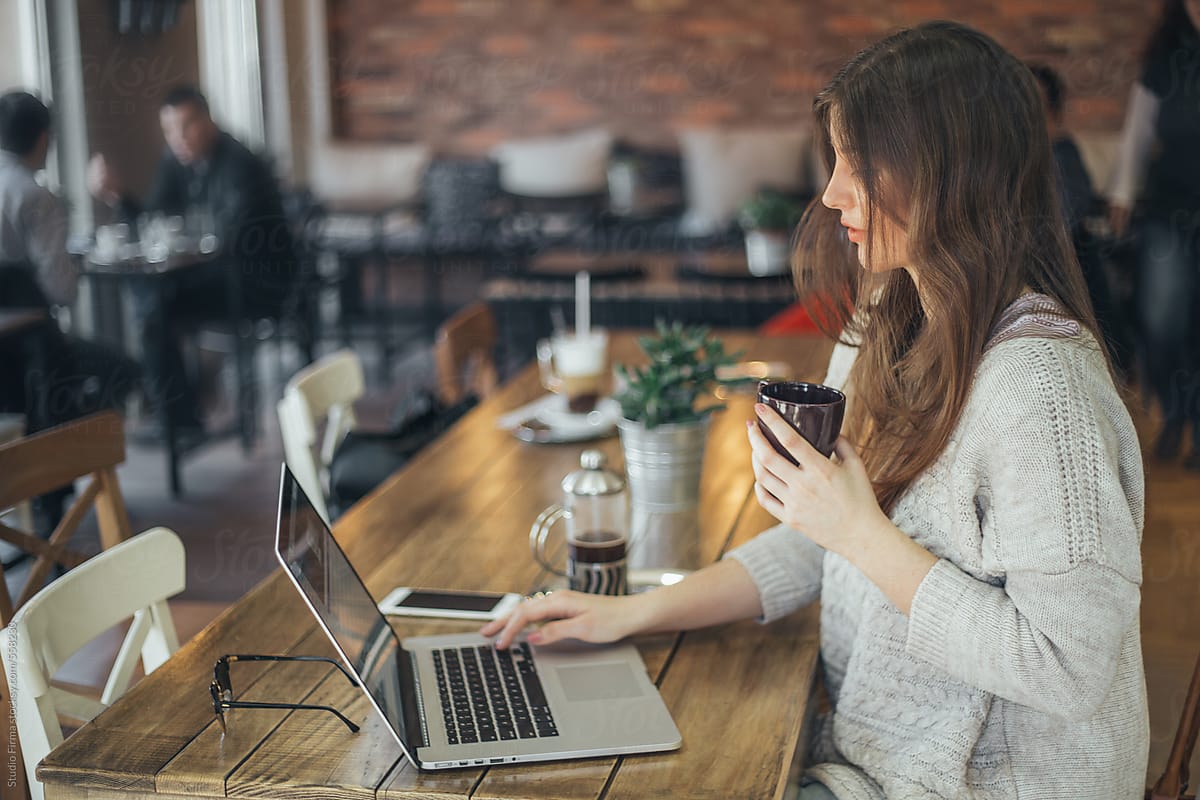
In the era of digitalization, producing insightful reports is essential to help businesses make wise decisions. One of the most widely used report formats, RDLC is compatible with ASP.NET applications. Still, no updates have been made to enable it to function properly with ASP.NET Core. Bold Reports provides a Report Viewer that you can incorporate into your ASP.NET Core applications in order to fulfill this requirement and display RDLC reports without having to switch to a different reporting system. We’ll walk you through the process of integrating this Report Viewer and displaying your RDLC reports in your ASP.NET Core application in this blog.
Create a New ASP.NET Core Web Application
Let’s see how to create a new ASP.NET Core web application. I will be using Visual Studio 2022, but the steps should be similar for other versions of Visual Studio:
- First, open Visual Studio 2022. Then, click on the Create new project button.
- In the Create new project dialog, select the ASP.NET Core Web Application (Model-View-Controller) template. Then, click Next.
- In the Configure your new project dialog, change the project name, and then click Next.
- In the Additional information dialog, set the Target framework to .NET 6.0. Then, click Create.
Additional Information Dialog
The new ASP.NET Core web application will be created.
NoteThe Bold Reports ASP.NET Core Report Viewer works in ASP.NET Core 2.x, ASP.NET Core 3.x, and ASP.NET Core 5.0 versions.
Install NuGet Packages
In this section, we will install the necessary NuGet packages in the application. To install the NuGet packages, follow these steps:
- In the Solution Explorer tab, right-click the project and choose Manage NuGet Packages.
Choose Manage NuGet Packages
- In the Browse tab, search for the following packages:
- BoldReports.AspNet.Core—Creates a client-side reporting control using tag helpers.
- BoldReports.Net.Core—Creates a Web API service to process the reports.
- System.Data.SqlClient—This is an optional package. It should be installed if the RDL report contains an SQL Server or SQL Azure data source.
- Click Install to install the packages. The dependent packages will be installed automatically when installing the BoldReports.Net.Core package.
Referencing Scripts and CSS
To render the Report Viewer control, we need to reference the scripts and CSS. In this blog, we will use CDN links to reference scripts and a theme. However, you can also use local scripts and themes. Follow these steps to add the necessary references:
- Open the _Layout.cshtml page in the Views\Shared folder.
Layout.cshtml Page - Copy the following scripts and add them inside the <head> tag.
<link href="https://cdn.boldreports.com/5.1.20/content/material/bold.reports.all.min.css" rel="stylesheet" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <!--Render the gauge item. Add this script only if your report contains the gauge report item. --> <script src="https://cdn.boldreports.com/5.1.20/scripts/common/ej2-base.min.js"></script> <script src="https://cdn.boldreports.com/5.1.20/scripts/common/ej2-data.min.js"></script> <script src="https://cdn.boldreports.com/5.1.20/scripts/common/ej2-pdf-export.min.js"></script> <script src="https://cdn.boldreports.com/5.1.20/scripts/common/ej2-svg-base.min.js"></script> <script src="https://cdn.boldreports.com/5.1.20/scripts/data-visualization/ej2-lineargauge.min.js"></script> <script src="https://cdn.boldreports.com/5.1.20/scripts/data-visualization/ej2-circulargauge.min.js"></script> <!--Render the map item. Add this script only if your report contains the map report item.--> <script src="https://cdn.boldreports.com/5.1.20/scripts/data-visualization/ej2-maps.min.js"></script> <script src="https://cdn.boldreports.com/5.1.20/scripts/common/bold.reports.common.min.js"></script> <script src="https://cdn.boldreports.com/5.1.20/scripts/common/bold.reports.widgets.min.js"></script> <!--Render the chart item. Add this script only if your report contains the chart report item.--> <script src="https://cdn.boldreports.com/5.1.20/scripts/data-visualization/ej.chart.min.js"></script> <!-- Report Viewer component script--> <script src="https://cdn.boldreports.com/5.1.20/scripts/bold.report-viewer.min.js"></script>
Configuring Script Manager
Next, we need to configure the script manager. Copy the following code and replace the code in the <body> tag. The bold script manager in the <body> element places the Report Viewer control initialization script in the webpage. To initialize the scripts properly, the Script Manager should be included at the end of the <body> element.
<body> <div style="min-height: 600px;width: 100%;"> @RenderBody() </div> @RenderSection("Scripts", required: false) <!-- Bold Reports script manager --> <bold-script-manager></bold-script-manager> </body>
Open the _ViewImports.cshtml file from the Views folder and add the following line to initialize the Report Viewer component with tag helper support.
@using BoldReports.TagHelpers @addTagHelper *, BoldReports.AspNet.Core
Initializing the Report Viewer
The next step is to initialize the Report Viewer. Open the index.cshtml file from the Home folder and remove the existing code. Replace it with the following code.
<bold-report-viewer id="viewer"></bold-report-viewer>
The id attribute specifies the unique name for the Report Viewer component. In this blog, we have assigned the value viewer to the id attribute.
Then, create a folder named Resources in the wwwroot folder of your application. This is where we will keep the RDLC reports.

Add the product-list.rdlc file to the Resources folder.
Configuring the Web API
The ASP.NET Core Report Viewer requires a web API service to process RDL, RDLC, and SSRS report files. Create a new file and name it ReportViewerController.cs inside the controller folder.

In the controller file, add the following using statement.
using System.IO; using BoldReports.Web.ReportViewer;
In the Route attribute, add the action placeholder, and remove the API controller attribute. Then, inherit IReportController.
[Route("api/[controller]/[action]")] public class ReportViewerController : Controller, IReportController { ... }
Replace the template code in the controller file with the following code.
[Route("api/[controller]/[action]")] public class ReportViewerController : Controller, IReportController { // Report Viewer requires a memory cache to store the information of consecutive client requests and // have the rendered Report Viewer information in server. private Microsoft.Extensions.Caching.Memory.IMemoryCache _cache; // IWebHostEnvironment used with sample to get the application data from wwwroot. private Microsoft.AspNetCore.Hosting.IWebHostEnvironment _hostingEnvironment; // Post action to process the report from server based json parameters and send the result back to the client. public ReportViewerController(Microsoft.Extensions.Caching.Memory.IMemoryCache memoryCache, Microsoft.AspNetCore.Hosting.IWebHostEnvironment hostingEnvironment) { _cache = memoryCache; _hostingEnvironment = hostingEnvironment; } // Post action to process the report from server based json parameters and send the result back to the client. [HttpPost] public object PostReportAction([FromBody] Dictionary<string, object> jsonArray) { //Contains helper methods that help to process a Post or Get request from the Report Viewer control and return the response to the control. return ReportHelper.ProcessReport(jsonArray, this, this._cache); } // Method will be called to initialize the report information to load the report with ReportHelper for processing. [NonAction] public void OnInitReportOptions(ReportViewerOptions reportOption) { } // Method will be called when reported is loaded with internally to start to layout process with ReportHelper. [NonAction] public void OnReportLoaded(ReportViewerOptions reportOption) { } //Get action for getting resources from the report. [ActionName("GetResource")] [AcceptVerbs("GET")] // Method will be called from Report Viewer client to get the image src for Image report item. public object GetResource(ReportResource resource) { return ReportHelper.GetResource(resource, this, _cache); } [HttpPost] public object PostFormReportAction() { return ReportHelper.ProcessReport(null, this, _cache); } }
Setting report path and service URL
To render the reports available in the application with the Report Viewer, you need to set the report-path and report-service-url properties. Replace the following code on your Report Viewer page:
- Open the Index.cshtml page.
- Set the report-path and report-service-url properties, as shown in the following.
<bold-report-viewer id="viewer" report-path="productlist.rdlc" report-service-url="/api/ReportViewer"> </bold-report-viewer> <script type="text/javascript"> </script>
- Save the file.
Assign Data
For RDLC reports, we must provide the data for the application report. We need to provide the DataSources with OnInitialize option for Report Viewer.
Create a class file named ProductList.cs in the application root folder for the Product List report. Copy the following code and paste it inside the ProductList class.
public class ProductList { public string ProductName { get; set; } public string OrderId { get; set; } public double Price { get; set; } public string Category { get; set; } public string Ingredients { get; set; } public string ProductImage { get; set; } public static IList GetData() { List<ProductList> datas = new List<ProductList>(); ProductList data = null; data = new ProductList() { ProductName = "Baked Chicken and Cheese", OrderId = "323B60", Price = 55, Category = "Non-Veg", Ingredients = "grilled chicken, corn and olives.", ProductImage = "" }; datas.Add(data); data = new ProductList() { ProductName = "Chicken Delite", OrderId = "323B61", Price = 100, Category = "Non-Veg", Ingredients = "cheese, chicken chunks, onions & pineapple chunks.", ProductImage = "" }; datas.Add(data); data = new ProductList() { ProductName = "Chicken Tikka", OrderId = "323B62", Price = 64, Category = "Non-Veg", Ingredients = "onions, grilled chicken, chicken salami & tomatoes.", ProductImage = "" }; datas.Add(data); return datas; } }
In the OnInitReportOptions method, place the following code.
public void OnInitReportOptions(ReportViewerOptions reportOption) { reportOption.ReportModel.ProcessingMode = ProcessingMode.Local; string basePath = Path.Combine(_hostingEnvironment.WebRootPath, "Resources"); string reportPath = Path.Combine(basePath, reportOption.ReportModel.ReportPath); FileStream fileStream = new FileStream(reportPath, System.IO.FileMode.Open, System.IO.FileAccess.Read); MemoryStream reportStream = new MemoryStream(); fileStream.CopyTo(reportStream); reportStream.Position = 0; fileStream.Close(); reportOption.ReportModel.Stream = reportStream; reportOption.ReportModel.DataSources.Add(new BoldReports.Web.ReportDataSource { Name = "list", Value = ProductList.GetData() }); }
Now everything is ready to render a RDLC report from the ASP.NET Core application. Build and run the application, and you will see the report is loaded in the browser.

Conclusion
The ASP.NET Core Report Viewer’s RDLC reporting feature enables developers to display data in an understandable and aesthetically pleasing way. You can give users thorough and interactive reports by utilizing the RDLC reporting engine’s power and seamlessly integrating it into ASP.NET Core applications. The ASP.NET Core Report Viewer with RDLC reporting is a powerful tool for data visualization, making it ideal for use in business analytics, financial statements, and other data-centric applications. To provide your users with reports that have an impact, explore and unleash the potential of RDLC reporting in your ASP.NET Core projects.